Django is one of the leading Python frameworks used to create full-stack web applications. In this comprehensive guide, you will explore the intricacies of testing and debugging within the Django framework, focusing on advanced methodologies and essential tools.
Beginning with the fundamentals of Django unit and integration testing, you will delve into advanced techniques such as mocking, testing middleware, and profiling for optimal performance. You will discover effective debugging strategies, from utilizing the Django Debug Toolbar to remote debugging with PyCharm or VS Code.
Django Testing Basics
Just as with any other framework/language, testing is a critical aspect of Django development. Robust testing ensures that your Django web applications function as intended and you catch potential issues early in the development process.
Django Unit Testing vs Integration Testing
Unit tests and integration tests both help lay down the foundation of a robust testing strategy for Django web apps. Still, understanding the differences between unit testing and integration testing is fundamental to effective testing in Django.
Django unit testing involves isolating individual components or functions and verifying that they work as expected in isolation. On the other hand, integration testing focuses on examining how different components or modules interact with each other. In Django, the unittest module provides a robust framework for both types of testing.
Django unit tests are typically faster and pinpoint specific issues within isolated parts of your codebase. Integration tests, meanwhile, ensure that different components work seamlessly together, catching issues that might not surface in unit tests alone. Striking a balance between these two types of tests is crucial for complete test coverage in your apps.
Writing and running basic Django tests
Writing tests in Django is a rather straightforward process, compared to some other frameworks.
To start, create a file within your Django app directory with a name like test_models.py, test_views.py, or test_forms.py, depending on the aspect of the app you want to test. Use the unittest module or Django’s built-in testing utilities, such as TestCase, to define your test cases.
For instance, here’s how a very basic Django unit test for a model class named “MyModel” which has an attribute “name” would look like:
from django.test import TestCase
from myapp.models import MyModel
class MyModelTestCase(TestCase):
def test_model_creation(self):
obj = MyModel.objects.create(name="Test Object")
self.assertEqual(obj.name, "Test Object")
This Django unit test creates an instance of the “MyModel” model and checks for data integrity. To run tests, you can use the python manage.py test command, which automatically discovers and executes all tests in your Django project.
Django test case classes and fixtures
Django provides specialized test case classes that offer additional features and utilities tailored for various testing scenarios. The django.test.TestCase class, which you saw in the example test case just now, provides a test database that is rolled back after each test, ensuring a clean slate for every test method.
Fixtures are another essential aspect of testing in Django. They represent pre-loaded data used for testing purposes. Django’s fixtures, often written in JSON or YAML format, enable you to populate your database with predefined data before running tests.
Here’s how you can define and use fixtures:
# In fixtures/my_fixture.json
[
{
"model": "myapp.mymodel",
"pk": 1,
"fields": {
"name": "Test Object"
}
}
]
# In your test case
class MyModelTestCase(TestCase):
fixtures = ['my_fixture.json']
def test_model_creation(self):
obj = MyModel.objects.get(pk)
self.assertEqual(obj.name, "Test Object")
Best practices for writing testable code
Writing testable code is essential for maintaining a robust testing suite. You can follow these best practices to enhance the testability of your Django applications:
- Separation of Concerns: Keep your code modular with a clear separation of concerns. Well-organized code is easier to test and maintain.
- Use Dependency Injection: Instead of hardcoding dependencies, inject them into your functions or classes. This allows for easier substitution of dependencies during testing.
- Avoid Global State: Global state can introduce unexpected behavior in tests. Minimize the use of global variables or stateful objects.
- Keep Tests Independent: Ensure that each test is independent of others. Avoid dependencies between tests to isolate issues when they arise.
- Use Test-Driven Development (TDD): Consider adopting TDD principles, where you write tests before implementing functionality. This approach encourages a focus on testable designs.
Advanced Django Test & Debug Techniques
As your Django project grows in complexity, mastering advanced testing techniques becomes crucial. In this section, you will learn some of the intricacies of mocking and patching, testing middleware and decorators, creating custom test runners and plugins, assessing Django REST framework APIs, and conducting performance testing to ensure your applications meet high standards.
Mocking and Patching in Django tests
Mocking and patching are powerful tools in your Django test toolkit. They enable you to simulate external dependencies or alter the behavior of specific functions or classes without affecting the actual implementation. The unittest.mock module in Python facilitates this process.
Consider a scenario where your Django application interacts with an external API. Instead of making actual HTTP requests during tests, you can mock the requests, and check if the external APIs were called. You could also return dummy responses from these APIs in your tests to ensure predictable and controlled testing environments.
from unittest.mock import patch
from django.test import TestCase
from myapp.services import external_api_call
class MyTestCase(TestCase):
def setUp(self):
self.service = Service()
@patch("my_view.fetch_from_external_api", autospec=True)
def test_api_interaction(self, mock_fetch_from_external_api):
self.service.get("/api/version")
self.assertTrue(mock_fetch_from_external_api.called)
The code above mocks a method fetch_from_external_api in the my_view module. Instead of calling the external API, the test code just checks if a call to the external API was made or not, indicating that the overlying service.get function worked as expected.
Custom Django test runners and plugins
Django’s default test runner is powerful, but in some cases, you might need more control over the testing process. Creating a custom Django test runner allows you to tailor the testing environment to your specific needs. This can include setting up additional databases, customizing output formats, or integrating with external testing tools.
Additionally, Django supports the use of plugins to extend testing capabilities. These plugins can add functionalities such as code coverage analysis, parallel test execution, or integration with external services.
Performance testing in Django Apps
Ensuring your Django application performs well under various conditions is crucial for delivering a delightful user experience. Performance testing in Django apps involves evaluating the speed, responsiveness, and stability of your application under different workloads.
Django is compatible with tools like pytest that integrate with performance-testing libraries. Additionally, profiling tools like django-silk can help identify performance bottlenecks in your codebase.
By incorporating these advanced testing techniques into your Django development workflow, you can build robust, efficient, and high-performance applications. In the next segments of this guide, we’ll explore debugging strategies, automation, and security testing to further elevate your Django development skills.
Built-in Profiling in Django
Django has a well-maintained companion app for profiling: django-debug-toolbar. This versatile tool not only provides insights into the queries executed but also allows for profiling views. You can get started with the tool by installing it using this command:
pip install django-debug-toolbar
Next, you will need to add the following to your INSTALLED_APPS and MIDDLEWARE settings in the settings.py file:
INSTALLED_APPS = [
# other apps
'debug_toolbar',
]
MIDDLEWARE = [
# other middleware
"debug_toolbar.middleware.DebugToolbarMiddleware",
]
Also, add this URL to your project’s URL config file:
from django.urls import include, path
urlpatterns = [
# ...
path("__debug__/", include("debug_toolbar.urls")),
]
Finally, configure the internal IP to show the toolbar on your machine:
INTERNAL_IPS = [
“127.0.0.1”,
]
That’s it. You can now run the development server and view the toolbar on the right hand side of the screen when developing the app.
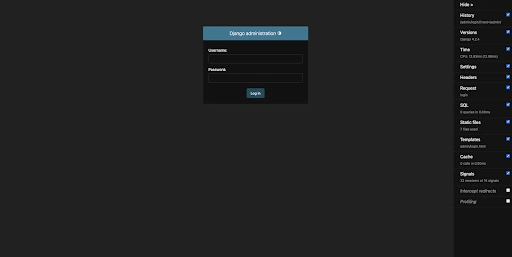
Clicking on any of the list items in the toolbar will open up the relevant screen, such as the Settings page:

You can view time-related metrics in the Time section of the toolbar, such as the time spent in each step of the rendering process and the resources used in it. You also get basic SQL queries analysis and static file statistics, along with templates, cache, and signals-related information.
Profiling Django using django-silk
django-silk is a popular open-source tool used for profiling Django apps. It provides detailed profiling of database queries, cache usage, and template rendering times. DJango-silk captures and logs every HTTP request and response, providing a detailed history of the application's interactions. It also includes a Query Explorer, allowing developers to interactively explore and analyze database queries made during a request. Furthermore, django-silk enables the replay of requests directly from the Django admin interface.
Here’s how you can get started with django-silk. First of all, install the package using the following command:
pip install django-silk
Then, in the settings.py file, add the following:
MIDDLEWARE = [
...
'silk.middleware.SilkyMiddleware',
...
]
INSTALLED_APPS = (
...
'silk'
)
SILKY_PYTHON_PROFILER = True
In your project’s urls.py, add the following:
urlpatterns = [
# Add the following path
path('silk/', include('silk.urls', namespace='silk'))
]
Make sure to run migrations before running the project (using the python manage.py migrate command).
Now when you run the app, silk will automatically start intercepting requests and you can begin with profiling. You can access the silk interface at /silk route. Here’s what it looks like for a fresh Django app with just one view:

You will need to use the silk_profile decorator or context manager to profile sections of your code. For instance, here’s a simple view instrumented with the silk_profile context manager:
from django.http import HttpResponse
from silk.profiling.profiler import silk_profile
import time
def index(request):
with silk_profile(name="slow request test"):
print("going to sleep")
time.sleep(3)
print("woke up")
return HttpResponse("Hello, world. You're at the polls index.")
After you run this and look into the requests tab at /polls, you will see the following:
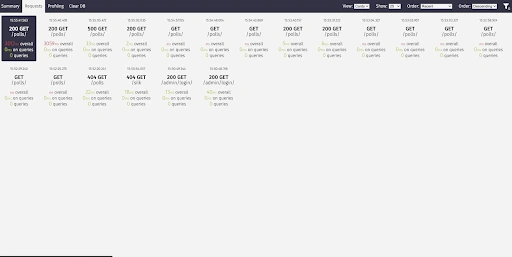
Click on the first requests and go to the profiling tab:

You will find a list of all the profiling instances attempted in this request:

Once you click on an instance, you will see more details, such as the piece of code that was profiled and the dump from the cPython profiler for that piece of code:
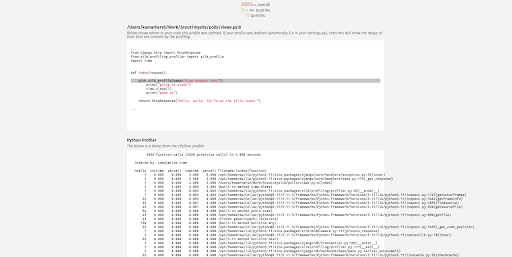
This is how you can use the django-silk profiler to easily profile your Django apps!
Django testing & debugging: Conclusion
In this guide, we’ve delved into the domain of testing and debugging within the Django framework, exploring both foundational principles and advanced techniques. From mastering the basics of Django unit and integration testing to harnessing the power of mocking and patching, we’ve equipped Django developers with a robust strategy to ensure the reliability and functionality of their applications.
By integrating these advanced Django testing strategies and tools into your development workflow, you not only fortify the quality of your code but also streamline the Django debugging process. The journey doesn’t end here; in addition to in-depth monitoring like you get with Scout APM, testing is a critical part of fortifying your applications in the evolution of your Django development skills.